
Table of Contents
Introduction to Python: The Beginner’s Guide to a Powerful Programming Language
Python has become one of the most popular and versatile programming languages in the world. Whether you’re new to programming or looking to expand your skills, learning Python can open up countless opportunities in web development, data science, machine learning, automation, and more. In this Introduction to Python, we’ll explore what Python is, why it’s so widely used, and how you can get started.
1. What is Python?
Python is a high-level, interpreted programming language known for its simplicity and readability. Created by Guido van Rossum in 1991, Python was designed to emphasize code readability and reduce the complexity of writing programs. Its easy-to-learn syntax makes it an ideal choice for both beginners and experienced developers.
2. Why is Python So Popular?
Python’s popularity has soared in recent years due to its versatility and ease of use. Here are some reasons why developers love Python:
- Simplicity: Python has an English-like syntax that makes it easy to read and understand, allowing developers to focus on solving problems rather than getting bogged down by complex code structures.
- Large Community: Python has a vast and active community, meaning there are plenty of resources, libraries, frameworks, and tutorials available for anyone looking to learn.
- Versatility: Python can be used for web development, data analysis, automation, artificial intelligence (AI), machine learning (ML), and more.
- Cross-Platform: Python is cross-platform, meaning it works on various operating systems like Windows, macOS, and Linux.
3. Key Features of Python
Python is known for several key features that make it stand out from other programming languages:
- Interpreted Language: Python is executed line by line, which makes debugging easier for beginners.
- Dynamic Typing: You don’t need to declare variable types in Python; it figures out the data type automatically, reducing the complexity of the code.
- Extensive Standard Library: Python’s standard library includes modules for everything from handling web requests to working with files, making it an incredibly versatile language.
- Support for Multiple Paradigms: Python supports procedural, object-oriented, and functional programming, offering flexibility in how you approach problem-solving.
- Third-Party Libraries: There are thousands of third-party libraries available for Python, such as NumPy and Pandas for data analysis, Django for web development, and TensorFlow for machine learning.
4. What Can You Do with Python?
Python’s wide range of applications makes it a must-learn language for developers of all levels. Here are some of the areas where Python excels:
1. Web Development
Python frameworks like Django and Flask are used to build powerful, scalable web applications. Django is known for its robustness, while Flask is a lightweight framework suited for smaller projects.
2. Data Science and Machine Learning
Python is the go-to language for data scientists and machine learning engineers. Libraries like Pandas for data manipulation, NumPy for numerical computations, and Matplotlib for data visualization make it easy to analyze data. Python also has excellent machine learning libraries such as scikit-learn and TensorFlow.
3. Automation and Scripting
Python is frequently used for automating repetitive tasks, such as file management, web scraping, and testing. With just a few lines of code, you can create scripts that save you hours of manual work.
4. Game Development
Though not as common as other languages for gaming, Python’s library Pygame allows for the creation of simple 2D games. It’s an excellent way for beginners to understand game development concepts.
5. Artificial Intelligence and Deep Learning
Python is at the forefront of AI and deep learning, with frameworks like TensorFlow, Keras, and PyTorch enabling the development of AI models, neural networks, and advanced algorithms.
6. Desktop Application Development
Python can also be used to build desktop applications with libraries like Tkinter and PyQt, making it possible to create GUI-based applications.
5. Getting Started with Python
Ready to dive in? Here’s how to get started with Python:
Step 1: Install Python
- Visit the official Python website (https://python.org) and download the latest version.
- Install it on your machine, ensuring that you check the option to add Python to your PATH during installation.
Step 2: Write Your First Python Program
After installing Python, you can write your first program. Open a text editor or the built-in IDLE (Python’s Integrated Development Environment), and type the following code:
print("Hello, World!")
Save the file with a .py
extension and run it in your terminal or command prompt by typing python filename.py
.
Step 3: Learn the Basics
Begin by learning the fundamentals of Python, such as:
- Variables and Data Types: Learn how to store data in variables and work with different data types like integers, strings, and floats.
- Control Flow: Understand how to use conditionals (
if
,else
,elif
) and loops (for
,while
) to control the flow of your program. - Functions: Learn how to write reusable blocks of code using functions.
- Lists, Tuples, and Dictionaries: These are essential data structures in Python that allow you to store and manipulate collections of data.
Step 4: Explore Python Libraries
Once you are comfortable with the basics, start exploring popular Python libraries that match your area of interest. For example:
- Pandas for data manipulation
- Django for web development
- OpenCV for computer vision
- BeautifulSoup for web scraping
Step 5: Practice, Practice, Practice!
The best way to learn Python is through hands-on practice. Use platforms like LeetCode, HackerRank, and Codecademy to solve problems and enhance your skills.
6. Why Python is a Great First Language
Python is often recommended as the first programming language for beginners due to its simple and readable syntax. Unlike other languages, Python’s syntax is clean and easy to follow, making it easier to grasp fundamental programming concepts. Additionally, Python’s widespread usage in multiple fields ensures that what you learn can be applied across a wide range of industries.
Why Learn Python?
Python is a powerful and versatile language that opens up endless possibilities in the world of technology. Whether you’re interested in web development, data science, artificial intelligence, or automation, Python has the tools and libraries to help you succeed.
Its clean syntax, vast library support, and strong community make it an excellent language for beginners while offering advanced features that keep experienced developers engaged. By learning Python, you’ll be equipped with a skill set that is highly in demand in today’s tech-driven world.
Ready to start your journey with Python? Now is the perfect time to get hands-on with this versatile language and unlock new career opportunities!

How Python is Used: Exploring the Versatility of Python Programming
Python has rapidly grown into one of the most widely used programming languages across a variety of industries. Its simplicity, flexibility, and extensive libraries have made it the go-to choice for developers, data scientists, and engineers alike. In this article, we will explore how Python is used in different fields, from web development to artificial intelligence, and why it’s a must-learn language for anyone looking to build a career in tech.
1. Python for Web Development
One of the most common uses of Python is web development. With popular frameworks like Django and Flask, Python is used to build secure, scalable, and robust web applications.
- Django: This high-level Python framework encourages rapid development and clean, pragmatic design. It comes with built-in features like an admin panel, authentication, and database management, which make it a great choice for building large-scale web applications.
- Flask: Unlike Django, Flask is a micro-framework that gives developers more control and flexibility. It’s lightweight, making it ideal for smaller projects or those requiring more customization.
Python’s ease of use, combined with these frameworks, allows developers to quickly create web applications, handle backend processes, and connect to databases.
2. Python in Data Science and Machine Learning
Python is the most popular language in data science and machine learning due to its powerful libraries and frameworks:
- Pandas: This library provides data structures and functions needed for manipulating numerical tables and time series data. It’s perfect for cleaning, analyzing, and visualizing datasets.
- NumPy: NumPy adds support for large multi-dimensional arrays and matrices. It’s essential for handling numerical data and performing mathematical computations.
- Matplotlib and Seaborn: These are Python libraries used for data visualization, helping you to create charts, graphs, and other visual representations of data.
- Scikit-learn: This is the most popular library for implementing machine learning algorithms like decision trees, random forests, and neural networks.
- TensorFlow and PyTorch: These are deep learning frameworks used to create advanced neural networks, making Python essential for AI and machine learning applications.
Data scientists and machine learning engineers use Python to build models, analyze data, and create systems that can learn and make predictions from large datasets.
3. Python in Automation and Scripting
Python’s simple syntax and powerful libraries make it an ideal choice for automation and scripting tasks. Whether you need to automate repetitive tasks like file management, send emails, or scrape data from websites, Python has a library for it:
- Selenium: Often used for browser automation and web scraping, Selenium is a popular Python tool for automating browser activities, such as testing web applications.
- BeautifulSoup: This library helps in extracting data from HTML and XML files. It’s frequently used for web scraping projects.
- OS Module: The OS module in Pithon allows for the interaction with the operating system to perform tasks such as file manipulation, reading and writing files, or executing system commands.
Python’s ability to automate manual processes saves time and increases efficiency, making it a favorite among system administrators and developers.
4. Python for Game Development
Though not as popular as C++ for gaming, Pithon is still widely used in game development, especially for creating simple games. The Pygame library is often used to build 2D games, and it provides features like handling input devices, managing audio, and rendering graphics.
Many beginners find Pithon useful for creating simple games because of its easy learning curve, allowing them to focus on learning game development concepts.
5. Python in Artificial Intelligence (AI) and Deep Learning
Python’s dominance in the world of artificial intelligence (AI) and deep learning is unparalleled. Libraries like TensorFlow, Keras, and PyTorch allow developers to build complex AI models, including deep learning neural networks.
Python’s versatility, ease of use, and strong community support make it the go-to language for building AI-driven applications like:
- Image recognition: Pithon is used to train neural networks to identify images and classify objects.
- Natural language processing (NLP): Pithon enables machines to understand and process human language.
- Chatbots and virtual assistants: AI systems like chatbots and virtual assistants rely heavily on Pithon for logic and decision-making.
With Pithon, developers can create advanced AI applications that can learn and adapt, mimicking human intelligence.
6. Python in Finance and FinTech
In the world of finance and FinTech, Pithon has become a key tool for analyzing financial data, automating trading strategies, and building financial models. Financial analysts and developers use Pithon for tasks such as:
- Predictive analytics: Pithon can be used to predict stock prices, evaluate risks, and build investment models using machine learning.
- Quantitative trading: Developers create trading algorithms that execute trades based on market conditions or specific triggers.
- Risk management: Pithon is used to evaluate risks and automate financial processes that ensure compliance and accuracy.
Python’s ability to handle large datasets, combined with its machine learning capabilities, makes it invaluable for the financial industry.
7. Python in Scientific Computing
Pithon is heavily used in scientific computing and research fields due to its powerful mathematical libraries. Libraries such as SciPy and NumPy make it possible to perform complex scientific calculations, while Matplotlib allows researchers to visualize their findings.
Researchers in fields like physics, biology, and chemistry rely on Pithon for tasks such as:
- Simulation and modeling: Pithon is used to create simulations of natural systems or perform numerical experiments.
- Data analysis: Scientists can use Pithon to analyze large datasets from experiments and draw meaningful conclusions.
Python’s ability to process and analyze data quickly makes it an essential tool in scientific research.
The Endless Possibilities with Python
Pithon’s versatility makes it an incredibly powerful language across multiple industries. Whether you’re building websites, analyzing data, creating games, or developing AI models, Pithon provides the tools and libraries necessary to succeed. Its simplicity, extensive community support, and wide range of applications make Pithon a must-learn language for both beginners and experienced developers.
With Pithon, the possibilities are endless. If you’re looking to build a career in web development, data science, AI, or automation, learning Pithon will unlock countless opportunities and help you stay ahead in the ever-evolving tech landscape.
Are you ready to start your Pithon journey? Dive into this powerful language and explore its many applications!
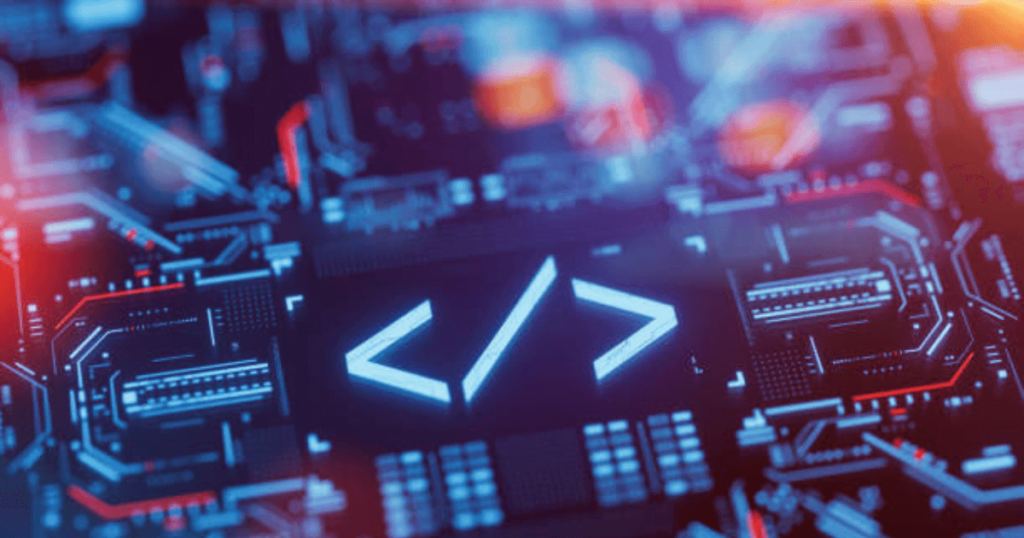
Why Pithon is Important: The Essential Programming Language for Modern Tech
Pithon has become one of the most important programming languages in today’s tech landscape. Its simple syntax, versatility, and powerful libraries have made it a preferred choice for developers across various industries, from web development to artificial intelligence (AI) and data science. In this article, we’ll explore why Python is important, its key features, and how it continues to shape the future of technology.
1. Pithon Simplicity and Ease of Learning
One of the most significant reasons why Pithon is important is its simplicity. Unlike other programming languages like Java or C++, Pithon syntax is clean, readable, and easy to learn. This makes it an ideal language for beginners who are new to programming and for seasoned developers who want to quickly prototype and build applications.
- Readable Code: Pithon syntax is designed to be almost like English, making it easy for developers to understand and write code without the need for complex brackets or semicolons.
- Minimal Boilerplate: Pithon eliminates unnecessary boilerplate code, reducing the amount of repetitive tasks and allowing developers to focus on solving real-world problems.
The ease of learning Pithon has led to its widespread adoption in schools, universities, and coding bootcamps, helping students and professionals transition into programming roles quickly.
2. Versatility Across Multiple Industries
Pithon versatility is another reason it’s important in the modern tech ecosystem. From web development to machine learning and data analysis, Pithon is used in almost every field, making it a must-learn language for developers and engineers.
Popular Pithon Applications Include:
- Web Development: With frameworks like Django and Flask, Pithon is used to build dynamic websites and web applications.
- Data Science and Machine Learning: Pithon libraries such as Pandas, NumPy, and scikit-learn make it the preferred language for data scientists and machine learning engineers.
- Automation and Scripting: Pithon is widely used for automating repetitive tasks, from file management to web scraping and testing.
- Game Development: Pithon libraries like Pygame allow for the development of simple 2D games.
Pithon ability to be used across different industries means that learning Pithon can open doors to various career paths, making it an important language for both beginners and experts.
3. Large Community and Extensive Libraries
Pithon large and active community plays a crucial role in its importance. As one of the most popular programming languages in the world, Pithon boasts a vast ecosystem of libraries, frameworks, and tools that make development easier and faster.
- Extensive Libraries: Pithon has thousands of libraries and modules available for specific tasks like data manipulation, machine learning, web development, and automation.
- Open-Source Support: The Pithon community continually contributes to open-source projects, ensuring that there are plenty of resources, tutorials, and tools available for developers of all levels.
- Problem Solving: Pithon active community ensures that there’s always help available. Whether you’re stuck on a problem or looking for best practices, forums like Stack Overflow and GitHub have vast resources to help you out.
The Pithon ecosystem’s richness and supportive community make it easier for developers to learn, grow, and solve complex problems efficiently.
4. Pithon in Data Science and Artificial Intelligence (AI)
Pithon has become the dominant language in the fields of data science and artificial intelligence due to its simplicity and vast number of powerful libraries. Here’s why Pithon is important in these cutting-edge fields:
- Data Analysis: Libraries like Pandas and NumPy allow data scientists to manipulate and analyze large datasets with ease, making Pithon the top choice for data analysis.
- Machine Learning: Pithon libraries such as scikit-learn, TensorFlow, and PyTorch are critical for building machine learning models that can process vast amounts of data, recognize patterns, and make predictions.
- AI Research: Pithon is at the forefront of AI research and development, providing the tools necessary to create neural networks, deep learning algorithms, and intelligent systems.
Pithon influence in these high-demand fields means that it’s not only important for developers but also for businesses looking to leverage AI and data science for innovation and growth.
5. Pithon is Cross-Platform and Open-Source
Another reason Pithon is so important is its cross-platform compatibility. Pithon can run on various operating systems, including Windows, macOS, and Linux. This flexibility means that developers can write code once and deploy it across different environments without significant changes.
- Open-Source Nature: Pithon is open-source, meaning that it is free to use, modify, and distribute. This accessibility has helped it gain traction worldwide, particularly in educational institutions and among startup communities.
The open-source nature and cross-platform capabilities of Pithon make it a reliable and future-proof language for developers looking to build software that works across multiple systems.
6. Pithon Role in Automation
In today’s fast-paced world, automation is crucial for increasing productivity and reducing manual tasks. Pithon excels in automation, thanks to its straightforward syntax and powerful automation libraries such as:
- Selenium: For browser automation and web testing.
- BeautifulSoup: For web scraping and data extraction.
- OS and Shutil: For file handling and system automation.
By using Pithon for automation, businesses can save time, reduce human error, and optimize their workflows. This makes Python a key language for companies looking to streamline operations.
7. Pithon Impact on Career Growth
Learning Pithon can significantly boost your career prospects. As one of the top programming languages used by leading tech companies like Google, Facebook, and Netflix, Python skills are highly sought after by employers across various sectors.
- High Demand: Pithon is consistently ranked among the most in-demand programming languages. Industries ranging from finance to healthcare are actively seeking Pithon developers to work on innovative projects.
- High Salary: Pithon developers typically earn competitive salaries, with many positions offering six figures. Mastering Python can lead to roles in software development, data analysis, machine learning, and more.
The increasing demand for Pithon in the job market makes it a vital skill for anyone looking to pursue a career in tech.
Why Pithon is Important
Pithonsimplicity, versatility, and powerful ecosystem have made it an essential programming language for developers and businesses alike. Whether you’re a beginner looking to enter the world of programming or a professional seeking to expand your skill set, learning Pithon can provide you with countless opportunities.
From web development to data science, machine learning, and automation, Pithon is at the core of many modern technologies shaping the future. Its growing influence across industries means that mastering Pithon will not only enhance your coding abilities but also unlock new career paths in an ever-evolving tech landscape.
Start learning Pithon today and harness the full potential of one of the most important programming languages in the world!
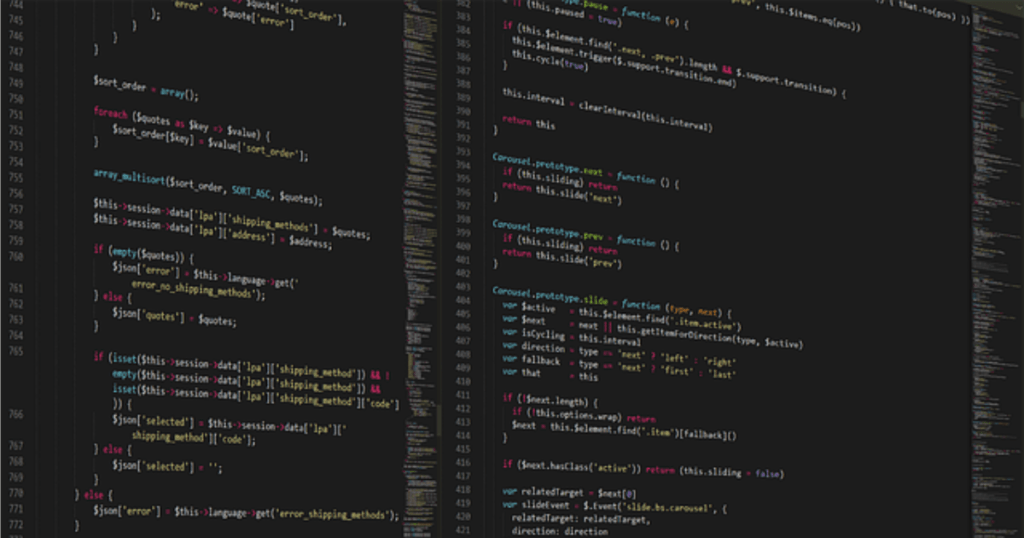
10 Effective Tips to Learn Pithon Fast: Accelerate Your Coding Journey
Pithon has become one of the most popular programming languages due to its simplicity, readability, and versatility. Whether you’re diving into web development, data science, or automation, Python is an essential skill. But how do you learn Python quickly and efficiently? In this article, we’ll share 10 effective tips to help you learn Python fast and kickstart your journey to becoming a proficient programmer.
1. Start with the Basics: Master Pithon Fundamentals
Before jumping into advanced concepts, it’s crucial to master the basics of Pithon. Focus on understanding:
- Syntax: Learn how Pithon syntax works, including indentation, variables, and data types (strings, integers, and floats).
- Basic Operations: Practice basic operations such as arithmetic, comparison, and logical operators.
- Control Structures: Learn to use if-else statements, for and while loops, and functions to control the flow of your program.
Once you’re comfortable with the fundamentals, you’ll find it easier to progress to more complex topics.
2. Practice Coding Every Day
Consistency is key when learning Pithon (or any programming language). Set aside time to code every day, even if it’s just 30 minutes. Regular practice helps you retain what you’ve learned and solidifies your understanding of concepts.
How to Stay Consistent:
- Set daily or weekly goals: Challenge yourself to complete a small project or learn a new topic each week.
- Use coding platforms: Websites like LeetCode, HackerRank, and Codewars offer coding challenges that can help you practice regularly.
3. Break Problems into Smaller Steps
When working on a coding problem, it’s easy to feel overwhelmed. A key strategy is to break problems into smaller, manageable steps. This not only helps you focus on solving one part at a time but also improves your problem-solving skills.
Example:
If you’re asked to write a Pithon program that analyzes text, start by writing code to:
- Read the input text.
- Count the number of words.
- Identify specific keywords or patterns. By tackling each step individually, you’ll feel less overwhelmed and can progress steadily.
4. Learn by Doing Projects
One of the most effective ways to learn Pithon fast is by building real-world projects. When you create projects, you apply what you’ve learned, encounter new challenges, and reinforce your coding skills.
Examples of Simple Projects to Build:
- Calculator: Create a Python-based calculator with basic arithmetic operations.
- To-Do List: Build a simple command-line to-do list where users can add and remove tasks.
- Weather App: Use an API to fetch and display weather data for a specific city.
By working on projects, you gain hands-on experience that accelerates your learning.
5. Take Advantage of Online Resources
There are countless free and paid resources online to help you learn Pithon quickly. These resources cater to different learning styles, whether you prefer reading, watching videos, or engaging with interactive content.
Recommended Pithon Learning Resources:
- Python.org: Official documentation and tutorials for Pithon.
- Real Pithon: Offers detailed Pithon tutorials and coding tips.
- Codecademy: Interactive Pithon courses and projects.
- YouTube: Search for Pithon tutorials on channels like Corey Schafer or freeCodeCamp.
Diversifying your learning resources will help you grasp concepts from different perspectives and solidify your knowledge.
6. Understand Error Messages
When learning Pithon, you’ll inevitably encounter errors and bugs. Understanding error messages is key to debugging your code efficiently. Instead of feeling frustrated, use errors as learning opportunities.
- Read the error message carefully: Pithon provides detailed error messages that can help you identify where and why the error occurred.
- Search for solutions online: If you’re stuck, platforms like Stack Overflow have answers to almost every error you might encounter.
By becoming comfortable with error messages, you’ll be able to fix your code faster and learn from your mistakes.
7. Join Pithon Communities
Learning Pithon doesn’t have to be a solo journey. Joining Pithon communities allows you to ask questions, share knowledge, and stay motivated.
Popular Pithon Communities to Join:
- Stack Overflow: A great platform to ask questions and get help from experienced developers.
- Reddit’s r/learnpython: A community where beginners and experienced coders share tips, projects, and resources.
- GitHub: Contribute to open-source Pithon projects or collaborate with other developers to build your skills.
Engaging with others helps you stay accountable and provides access to a wealth of knowledge.
8. Use Pithon Libraries
One of Pithon biggest advantages is its vast ecosystem of libraries and frameworks that make development faster. Instead of writing everything from scratch, learn to use Pithon built-in libraries to save time.
Popular Pithon Libraries:
- NumPy: For numerical computing and data manipulation.
- Pandas: For data analysis and manipulation.
- Requests: For making HTTP requests and working with APIs.
- Matplotlib: For creating visualizations and graphs.
Learning to work with libraries will accelerate your development and broaden the scope of your projects.
9. Test Your Code Regularly
When learning Pithon, it’s important to test your code as you go along. Writing and testing code incrementally ensures that you catch bugs early and understand how your code works step by step.
Best Practices for Testing:
- Write unit tests: Use Pithon built-in
unittest
framework to test small parts of your code. - Use print statements: Insert
print()
statements throughout your code to check the values of variables or see if certain blocks of code are executing as expected.
Testing regularly helps you avoid large bugs and ensures that your program works as intended.
10. Stay Patient and Keep Learning
Learning Pithon (or any programming language) can take time, especially if you’re new to coding. Stay patient, and don’t be afraid to make mistakes. The more you code, the more comfortable you’ll become with Pithon syntax and logic.
Remember to keep challenging yourself with new projects, explore advanced topics, and always look for ways to improve your coding skills. Pithon is a powerful language, and mastering it will open the door to endless opportunities in the tech industry.
Learn Pithon Fast with Consistency and Practice
By following these 10 effective tips, you’ll be well on your way to learning Pithon quickly and efficiently. Whether you’re aiming to build web applications, analyze data, or create automated scripts, Pithon flexibility makes it an invaluable tool for developers of all levels.
Start small, practice regularly, and build real-world projects to reinforce what you’ve learned. With dedication and the right approach, you’ll master Pithon in no time!
5 Bad Pithon Habits to Avoid: Improve Your Code Quality
Pithon is one of the most popular and user-friendly programming languages, but even experienced developers can develop bad coding habits over time. These habits can lead to inefficient, buggy, and harder-to-maintain code. Whether you’re a beginner or an expert, it’s essential to recognize and avoid these common Pithon pitfalls.
In this article, we’ll explore 5 bad Pithon habits to avoid, which will help you write cleaner, more efficient, and maintainable code.
1. Using Global Variables Excessively
Global variables can seem like a quick solution when you need to access data across multiple functions. However, overusing them can lead to unintended consequences, making your code harder to debug and maintain.
Why It’s a Bad Habit:
- Code readability: Global variables make it difficult for others (and yourself) to understand where data is being modified.
- Unintended side effects: Global variables can be changed anywhere in your program, making it hard to trace where a bug originated.
The Solution:
Instead of relying on global variables, use local variables within functions. If you need to share data between functions, consider passing arguments or returning values. You can also use class attributes or encapsulate your data within objects to avoid global scope pollution.
# Bad practice: Using global variables
x = 10
def update_value():
global x
x += 5
# Better practice: Using function parameters and returns
def update_value(x):
return x + 5
x = update_value(x)
2. Not Handling Exceptions Properly
Many beginners (and even some experienced developers) often neglect error handling or catch all exceptions using broad exception clauses like except Exception
. This can lead to silent failures, where your program doesn’t crash, but bugs remain hidden.
Why It’s a Bad Habit:
- Hides errors: By catching all exceptions, you might ignore important errors that need to be addressed.
- Harder to debug: When you don’t know what went wrong, it becomes challenging to fix the problem.
The Solution:
Be specific when handling exceptions. Use targeted exception handling for expected errors and always log or report the error to help with debugging.
# Bad practice: Catching all exceptions
try:
# some code
except Exception:
pass # Silently ignoring errors
# Better practice: Handling specific exceptions
try:
# some code
except ValueError as e:
print(f”ValueError occurred: {e}“)
except FileNotFoundError as e:
print(f”FileNotFoundError: {e}“)
3. Writing Inefficient Loops
Pithon loops are a powerful tool, but writing inefficient loops can significantly slow down your program’s performance, especially when dealing with large data sets. A common mistake is to write loops where Pithon built-in functions or list comprehensions would be more efficient.
Why It’s a Bad Habit:
- Performance issues: Inefficient loops can lead to slower execution times, especially in data-intensive tasks.
- Readable code: Loops that can be replaced with built-in functions or comprehensions make the code longer and harder to read.
The Solution:
Use Pithon powerful built-in functions like map()
, filter()
, or list comprehensions to make your code more efficient and readable.
# Bad practice: Inefficient loops
squares = []
for i in range(10):
squares.append(i ** 2)
# Better practice: Using list comprehension
squares = [i ** 2 for i in range(10)]
4. Neglecting Code Readability and Comments
While Pithon syntax is designed to be readable, developers often overlook the importance of comments and code organization. This can make your code difficult to understand, especially when returning to it after a long time or when working with a team.
Why It’s a Bad Habit:
- Hard to maintain: Code without comments or clear structure can be challenging to update or debug.
- Team collaboration: If other developers can’t easily understand your code, it becomes difficult to collaborate or review the code effectively.
The Solution:
Follow PEP 8 (Pithon style guide) to format your code properly and use meaningful names for variables and functions. Add comments where necessary to explain complex logic, and consider using docstrings to document functions.
# Bad practice: No comments, unclear naming
def f(x):
return x ** 0.5
# Better practice: Descriptive naming and comments
def calculate_square_root(number):
“””Calculates the square root of a number.”””
return number ** 0.5
5. Ignoring Pythonic Principles
Pithon is known for its clear and concise syntax, often described as being “Pythonic.” However, many developers, especially those coming from other programming languages, tend to write code that doesn’t take full advantage of Pithon features.
Why It’s a Bad Habit:
- Unnecessarily complex code: Writing code that isn’t “Pythonic” leads to more complex, verbose, and harder-to-read code.
- Not leveraging Pithon strengths: Pithon has many built-in functions and language features designed to make code simpler and more efficient.
The Solution:
Familiarize yourself with Pythonic principles, such as using list comprehensions, context managers, and enumerations instead of more verbose alternatives. Writing Pythonic code improves readability and performance.
# Bad practice: Using traditional loop to enumerate
i = 0
for item in items:
print(i, item)
i += 1
# Better practice: Using Python. built-in enumerate function
for i, item in enumerate(items):
print(i, item)
Avoid These Bad Python. Habits for Cleaner, Efficient Code
By avoiding these 5 bad Python. habits, you can drastically improve your code quality, making it easier to read, maintain, and scale. Focus on writing readable, efficient, and Pythonic code, and always remember that good coding habits help you become a better programmer in the long run.
- Avoid excessive use of global variables.
- Handle exceptions properly with specific clauses.
- Write efficient loops and leverage built-in functions.
- Make your code readable with proper comments and formatting.
- Follow Pythonic principles to write concise and clear code.
Start applying these tips to your Python. projects today and see the difference in the quality of your code! home