
Table of Contents
What is JavaScript? A Complete Guide for Beginners
JavaScript is one of the most essential programming languages used for creating dynamic and interactive web content. It plays a crucial role in web development, enabling the creation of websites that are not just static pages but highly interactive platforms. Alongside HTML and CSS, JavaScript is one of the core technologies that power the web.
In this SEO-friendly article, we’ll explore what JavaScript is, how it works, and why it is so important for modern web development.
1. What is JavaScript?
JavaScript is a versatile, high-level programming language that was originally developed to add interactivity to websites. It allows web developers to create dynamic content, control multimedia, animate images, and much more. Over time, JavaScript has evolved from a client-side scripting language to a powerful tool used both on the client side (front-end) and the server side (back-end).
JavaScript enables developers to:
- Manipulate web content dynamically (e.g., form validation, dynamic menus)
- Create interactive websites (e.g., clickable buttons, pop-ups)
- Control multimedia elements like video and audio
- Fetch data from servers without reloading the entire page (via AJAX)
2. How JavaScript Works
JavaScript is typically embedded directly within an HTML document or linked as an external file. When a web browser loads the HTML file, it reads the JavaScript code, interprets it, and executes the instructions.
Basic JavaScript Example:
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Example</title>
<script>
function greetUser() {
alert('Hello, welcome to my website!');
}
</script>
</head>
<body>
<h1>Welcome to My Website</h1>
<button onclick="greetUser()">Click me</button>
</body>
</html>
In this example, a simple JavaScript function called greetUser()
is used to display an alert box when the button is clicked. This is a basic example of how JavaScript adds interactivity to a webpage.
How JavaScript is Embedded:
There are three primary ways to include JavaScript in a webpage:
- Inline JavaScript: JavaScript is written directly inside HTML elements using attributes like
onclick
. - Internal JavaScript: JavaScript is included inside the
<script>
tag in the HTML document’s<head>
or<body>
section. - External JavaScript: JavaScript code is written in a separate
.js
file and linked to the HTML document using the<script>
tag.
3. Why JavaScript is Important for Web Development
JavaScript is fundamental for modern web development. Here’s why:
1. Interactivity and User Experience:
JavaScript enhances the user experience by making websites more engaging and interactive. It allows users to interact with elements on a page without needing to refresh or reload the page. Examples include live chat widgets, slideshows, and dynamic form fields.
2. Client-Side Scripting:
JavaScript runs in the browser, meaning it can make quick updates to the page without needing a server response. This client-side scripting capability speeds up performance and improves the overall experience for users.
3. Asynchronous Communication:
With the help of technologies like AJAX (Asynchronous JavaScript and XML), JavaScript can send and retrieve data from a server in the background without disrupting the user experience. This allows for things like live content updates or loading new content without a page refresh (think social media feeds or live scores).
4. Cross-Browser Compatibility:
JavaScript works across all major browsers (Chrome, Firefox, Safari, Edge) without requiring special plugins, making it a universal choice for web developers.
5. Extensive Ecosystem:
JavaScript has a huge ecosystem, with countless libraries and frameworks such as React, Angular, and Vue.js. These tools help developers build complex applications more efficiently by providing pre-built code for common tasks.
4. JavaScript’s Role in Front-End and Back-End Development
Originally, JavaScript was only used on the client side to enhance user interaction in the browser. However, with the rise of technologies like Node.js, JavaScript is now also used on the server side to build scalable and efficient web applications.
JavaScript in Front-End Development:
In front-end development, JavaScript interacts with HTML and CSS to dynamically manipulate content, creating interactive user interfaces. Popular front-end frameworks include:
- React.js: Developed by Facebook, React is a JavaScript library used for building user interfaces, especially single-page applications.
- Vue.js: Vue is a progressive JavaScript framework used to build interactive web interfaces and single-page applications.
- Angular: Maintained by Google, Angular is a powerful framework for building complex front-end applications.
JavaScript in Back-End Development:
On the back-end, Node.js enables JavaScript to run on the server, handling requests and managing databases. This has transformed JavaScript into a full-stack language, capable of building both the front-end and back-end of web applications.
5. The Evolution of JavaScript
JavaScript has come a long way since its creation in 1995. Initially, it was considered a simple tool for adding minor interactivity to websites. However, with the development of ECMAScript standards (the official standardization of JavaScript), the language has grown to become much more powerful.
Modern JavaScript Features:
- Arrow Functions: A shorter syntax for writing functions.
- Promises and Async/Await: Tools for handling asynchronous operations, making code more readable and efficient.
- Modules: Ability to split JavaScript code into smaller, reusable files, improving code organization and maintainability.
- Classes: Object-oriented programming with a cleaner, more understandable syntax.
These updates have made JavaScript more developer-friendly and have allowed it to be used in increasingly complex projects.
6. Getting Started with JavaScript
If you’re new to JavaScript, the best way to get started is by practicing small examples and building simple projects. Here are some basic concepts to focus on as a beginner:
- Variables and Data Types: Learn how to store information using variables like
let
,const
, andvar
. - Functions: Understand how to write reusable code blocks using functions.
- Conditionals and Loops: Master basic logic using
if
statements,for
loops, andwhile
loops. - DOM Manipulation: Learn how to interact with the HTML document using JavaScript to add, remove, or modify content dynamically.
There are also many online resources, such as free JavaScript tutorials and code editors, to help you get started.
Why Learning JavaScript is Essential
JavaScript is a vital part of web development, powering everything from simple websites to complex web applications. Its versatility, ease of use, and extensive ecosystem make it the go-to language for both front-end and back-end development.
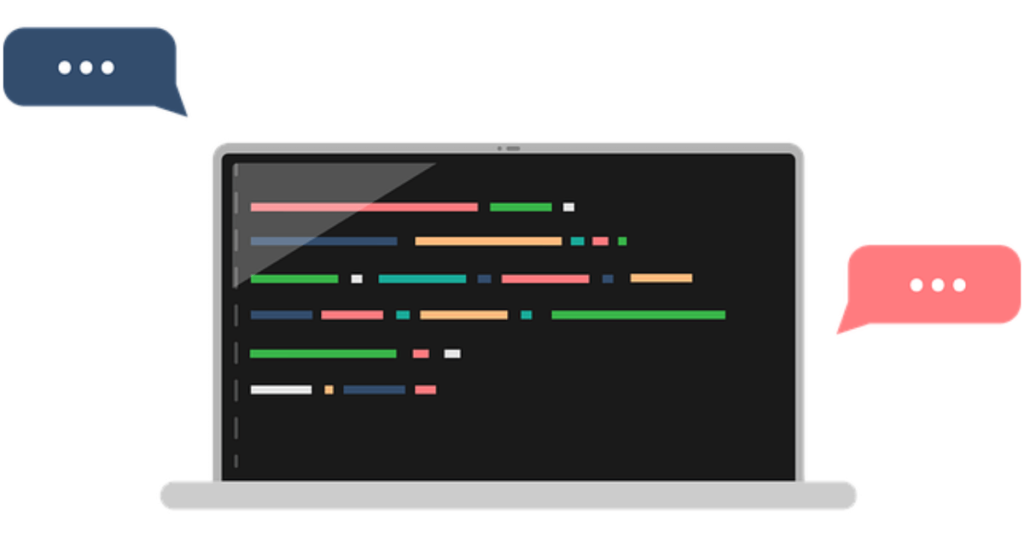
What Can You Do with Java.Script? A Comprehensive Guide
Java.Script is one of the most widely-used programming languages in the world, and it plays a pivotal role in web development. From creating interactive websites to building entire web applications, the versatility of JavaScript makes it an essential tool for developers. But beyond websites, what can you do with Java.Script? The answer is: a lot! This guide will explore the many different applications and possibilities of JavaScript and how it’s transforming industries.
1. Enhance Websites with Interactive Elements
At its core, JavaScript was initially designed to make websites more interactive. By integrating JavaScript with HTML and CSS, you can create engaging user experiences that go beyond static web pages.
Examples of Interactive Website Features:
- Form Validation: Use JavaScript to ensure that form fields are filled out correctly before submission.
- Sliders and Carousels: Create image sliders or carousels that change content dynamically.
- Pop-Ups and Modals: Trigger pop-ups, modals, and tooltips in response to user actions like clicking a button.
- Real-Time Updates: Display real-time data updates, such as live sports scores or stock prices, without refreshing the entire page.
By making websites more dynamic and user-friendly, JavaScript improves the overall user experience, which can lead to higher engagement rates.
2. Build Web Applications
JavaScript’s power extends far beyond small-scale websites. It’s the backbone of single-page applications (SPAs) and full-scale web applications. Frameworks and libraries like React.js, Vue.js, and Angular have made it easier than ever to develop robust web apps using JavaScript.
Examples of Web Apps You Can Build with JavaScript:
- Social Media Platforms: Websites like Facebook, Instagram, and Twitter rely heavily on JavaScript to manage front-end interactions.
- E-commerce Platforms: Build interactive shopping experiences with real-time product filtering, cart updates, and smooth navigation.
- Productivity Tools: Online applications like Trello or Google Docs are built using JavaScript, allowing real-time collaboration and task management.
JavaScript enables developers to build fast, responsive, and feature-rich applications that run seamlessly in the browser.
3. Create Mobile Apps
Did you know you can build mobile applications using JavaScript? With tools like React Native and Ionic, developers can create mobile apps for both iOS and Android platforms using the same JavaScript codebase.
Benefits of Using JavaScript for Mobile Apps:
- Cross-Platform Development: You can write one codebase and deploy it to both Android and iOS devices, saving time and resources.
- Native-Like Performance: Frameworks like React Native allow JavaScript to interact with native components, ensuring smooth performance.
- Access to Device Features: JavaScript can access mobile device features such as the camera, GPS, accelerometer, and more.
By leveraging JavaScript for mobile app development, businesses can launch their apps faster and reduce development costs.
4. Create Desktop Applications
JavaScript isn’t limited to the web or mobile devices. With platforms like Electron.js, developers can use JavaScript to build cross-platform desktop applications that run on Windows, macOS, and Linux.
Examples of Desktop Applications Built with JavaScript:
- Visual Studio Code: A popular code editor created by Microsoft, built using Electron.
- Slack: The widely-used messaging app for teams was also built using Electron and JavaScript.
- Spotify Desktop App: Spotify’s desktop app runs on Electron, taking advantage of JavaScript’s versatility.
By using JavaScript to develop desktop apps, developers can create rich, native-like experiences for users across multiple operating systems.
5. Server-Side Development with Node.js
JavaScript isn’t just for front-end development anymore. With the advent of Node.js, JavaScript can now be used for server-side development as well. This allows developers to build full-stack applications using a single programming language across both the client and server sides.
Benefits of Server-Side JavaScript:
- High Performance: Node.js is built on Google Chrome’s V8 engine, offering fast performance for I/O-heavy applications.
- Scalability: Node.js is ideal for building scalable network applications, such as chat apps and streaming services.
- Unified Codebase: Using JavaScript on both the front-end and back-end reduces complexity and improves collaboration among development teams.
Examples of applications using Node.js include Netflix, Uber, and LinkedIn, all of which rely on JavaScript to power their server-side operations.
6. Game Development
JavaScript can also be used to build 2D and 3D games that run directly in the browser. Tools like Phaser.js and Three.js make it easy to create engaging games with rich visuals and interactivity.
Examples of JavaScript in Game Development:
- Browser-Based Games: Build simple, browser-friendly games like puzzles, arcade games, or platformers using HTML5 Canvas and JavaScript.
- 3D Games: Create immersive 3D experiences with the help of libraries like Three.js, allowing for game development with realistic graphics.
With WebGL (Web Graphics Library), JavaScript can handle complex animations and render graphics directly in the browser, making it a viable option for game development.
7. Machine Learning and Artificial Intelligence
While JavaScript might not be the first language that comes to mind for machine learning or artificial intelligence, it’s gaining ground in these areas as well. Libraries like TensorFlow.js allow developers to build and run ML models directly in the browser or Node.js environment.
Examples of JavaScript in AI:
- Image Recognition: Build models that can recognize and classify images directly in a web browser.
- Natural Language Processing: Create chatbots or sentiment analysis tools using JavaScript-based machine learning models.
- Data Analysis: Use JavaScript to process and visualize large datasets in the browser.
JavaScript’s flexibility makes it a growing option for developers interested in integrating machine learning and AI capabilities into web-based applications.
8. Automation and Scripting
JavaScript can be used to automate tasks and create scripts that enhance productivity. With tools like Puppeteer or PhantomJS, JavaScript can control browsers and automate repetitive tasks like data scraping, form submissions, or screenshot generation.
Popular Uses of JavaScript for Automation:
- Web Scraping: Automate the extraction of data from websites.
- Testing: Run automated UI tests for web applications.
- Task Automation: Create scripts that automate daily tasks, such as logging into websites or filling out forms.
These capabilities make Java.Script a valuable tool for increasing productivity and reducing the need for manual intervention.
9. Internet of Things (IoT)
With the rise of the Internet of Things (IoT), Java.Script is being used to control and interact with IoT devices. Platforms like Node-RED and Johnny-Five enable developers to write Java.Script code that can control hardware like sensors, LEDs, and motors.
Examples of Java.Script in IoT:
- Smart Home Devices: Use Java.Script to control devices like lights, thermostats, or cameras.
- Wearables: Develop applications that run on wearable devices and interact with health data or fitness trackers.
JavaScript’s real-time capabilities make it ideal for building IoT applications that require fast and responsive interactions between devices.
Unlock Endless Possibilities with Java.Script
Java.Script is an incredibly versatile language that goes far beyond just making websites interactive. Whether you’re building web apps, mobile apps, desktop applications, or even venturing into machine learning and IoT, Java.Script offers a robust solution.

Where Does Java.Script Code Run? A Complete Guide for Beginners
Java.Script is one of the most widely-used programming languages in the world of web development. It allows developers to create interactive and dynamic features for websites and applications. But a common question for beginners is, where does Java.Script code actually run? In this article, we’ll explore the environments where Java.Script operates, how it works on both the client-side and server-side, and why understanding this is crucial for developers.
1. Java.Script in the Browser (Client-Side)
Historically, Java.Script was designed to run in web browsers. This is known as client-side Java.Script, which means that the code is executed on the user’s device (laptop, desktop, tablet, or smartphone) via their web browser. When you visit a website, the Java.Script code embedded in the webpage is sent to your browser, and it runs locally on your machine.
How Java.Script Works in the Browser:
- User Visits a Website: The browser loads the website’s HTML, CSS, and Java.Script files.
- Java.Script is Executed: Once the HTML is rendered, the browser executes the Java.Script code to make the page interactive (e.g., adding animations, validating forms, or handling button clicks).
- User Interacts: The browser responds to the user’s actions by running Java.Script to change or update parts of the page without reloading.
Examples of Client-Side Java.Script:
- Form Validation: Java.Script checks if form fields are filled correctly before submission.
- Interactive Content: It enables dynamic content like image sliders, animations, or real-time updates.
- DOM Manipulation: Java.Script can modify HTML and CSS, updating the user interface based on user input.
Popular browsers like Google Chrome, Mozilla Firefox, Safari, and Microsoft Edge all have built-in Java.Script engines that interpret and run Java.Script code.
2. Java.Script on the Server (Server-Side with Node.js)
While Java.Script was originally a client-side language, it has evolved to run on servers as well. With the introduction of Node.js in 2009, Java.Script can now be used for server-side development. This means that Java.Script code runs on the server, handling requests from clients, managing databases, and generating dynamic content before sending it back to the browser.
How Node.js Works:
- Single Language for Full-Stack: With Node.js, developers can use Java.Script on both the client and server sides, creating a unified environment for full-stack development.
- Event-Driven Architecture: Node.js is non-blocking and event-driven, meaning it can handle multiple requests simultaneously without slowing down.
- Server-Side Operations: Java.Script can manage tasks like reading files, connecting to databases, and serving content to users.
Examples of Server-Side Java.Script:
- APIs: Java.Script can handle API requests, fetching or sending data between the client and server.
- Real-Time Applications: Tools like Socket.io allow developers to create real-time apps (e.g., live chat applications) with Java.Script.
- Database Management: Java.Script can interact with databases like MongoDB, MySQL, and PostgreSQL to store or retrieve data.
3. The Browser’s Java.Script Engines
Each browser has its own Java.Script engine that is responsible for executing Java.Script code. These engines convert the Java.Script code into machine code that the device’s processor can understand and execute.
Popular Java.Script Engines:
- Google Chrome’s V8 Engine: This is one of the fastest and most widely-used Java.Script engines. It’s also the engine behind Node.js, enabling Java.Script to run on the server.
- Mozilla’s SpiderMonkey: This engine powers Firefox and has been optimized for efficient Java.Script execution.
- Safari’s JavaScriptCore (Nitro): Apple’s Java.Script engine for Safari, ensuring fast performance on Apple devices.
- Microsoft’s Chakra: Used by the Edge browser (before switching to Chromium), Chakra was designed for high-performance Java.Script execution.
Understanding that Java.Script engines are responsible for running the code is crucial, especially as developers work to optimize their applications for performance across different browsers.
4. How Java.Script Interacts with HTML and CSS
When Java.Script runs in the browser, it interacts with the Document Object Model (DOM), a representation of the HTML structure of a web page. Through Java.Script, developers can manipulate the DOM in real time, allowing for dynamic changes without requiring the user to reload the page.
Common Tasks Java.Script Performs:
- Changing Styles Dynamically: Java.Script can add or remove CSS classes to modify the appearance of elements based on user actions.
- Handling Events: Java.Script listens for events such as clicks, form submissions, or keyboard input and responds accordingly.
- Updating Content: Developers can use Java.Script to update the content of a webpage without making a new request to the server, improving performance and user experience.
5. Java.Script Outside the Browser: IoT, Game Development, and More
In addition to running in browsers and on servers, Java.Script is being used in other environments as well. Its versatility allows developers to use JavaScript in areas like:
Internet of Things (IoT):
With frameworks like Johnny-Five, Java.Script can control IoT devices such as lights, sensors, and motors. This makes it a valuable language for IoT development, allowing developers to create smart devices and applications.
Game Development:
Java.Script can also be used to develop games. With libraries like Phaser.js and Three.js, developers can create both 2D and 3D browser-based games. JavaScript’s integration with WebGL allows for complex graphics rendering, making it a viable option for game development.
Desktop Applications:
Tools like Electron.js allow developers to build cross-platform desktop applications using Java.Script. Popular apps like Visual Studio Code and Slack were built with Electron, demonstrating JavaScript’s power beyond web browsers.
6. Java.Script in Modern Development
In modern development, Java.Script plays a significant role across various platforms. The introduction of frameworks like React, Angular, and Vue has revolutionized how Java.Script is used in front-end development, while Node.js has made it a go-to language for back-end services.
Full-Stack Development:
With Java.Script running both in the browser (client-side) and on the server (server-side with Node.js), developers can use a single language to build entire web applications, streamlining the development process.
Real-Time Applications:
Java.Script is used to build real-time applications, such as chat apps, live streaming platforms, and collaborative tools. Libraries like Socket.io enable developers to handle real-time communication between clients and servers.
Where Does Java.Script Code Run?
Java.Script is an incredibly versatile language that runs in a variety of environments. Traditionally, JavaScript was a client-side language executed in the browser, but with the advent of Node.js, it has also become a powerful tool for server-side development. Additionally, JavaScript’s reach now extends into areas like desktop applications, game development, IoT, and even machine learning.
For developers looking to build interactive websites, full-scale web apps, mobile apps, or even IoT solutions, understanding where and how Java.Script runs is essential. Its flexibility and extensive ecosystem make JavaScript a cornerstone of modern software development, allowing it to function across multiple platforms and environments.

What is the Difference Between Java.Script and ECMAScript? A Clear Explanation
When diving into web development, you often hear terms like Java.Script and ECMAScript being used interchangeably. This can be confusing for beginners, leading many to wonder: what is the difference between Java.Script and ECMAScript? While these terms are closely related, they refer to different aspects of the same technology. In this article, we’ll explore the distinctions between Java.Script and ECMAScript, their history, and why understanding these differences is important for developers.
1. Java.Script: The Programming Language
Java.Script is a high-level, interpreted programming language used primarily for web development. It enables interactive elements on websites, such as form validation, dynamic content updates, and real-time interactivity. Java.Script is an essential tool for building modern web applications and can also be used in other areas like mobile app development and server-side programming.
Key Uses of Java.Script:
- Client-Side Interactions: Java.Script runs in the browser to manage dynamic web pages and user interactions.
- Server-Side Programming: With the introduction of Node.js, Java.Script is also used for building server-side applications.
- Mobile and Desktop Apps: Java.Script frameworks like React Native and Electron.js allow developers to create cross-platform mobile and desktop applications.
However, Java.Script is a language that implements a standard, and that standard is known as ECMAScript.
2. ECMAScript: The Language Standard
ECMAScript (often abbreviated as ES) is the specification or standard that defines how a scripting language like Java.Script should function. Created by the European Computer Manufacturers Association (ECMA), ECMAScript provides a blueprint that browser vendors and Java.Script engines follow to ensure compatibility and consistent behavior across different platforms.
Why ECMAScript Exists:
- Consistency Across Platforms: Before ECMAScript was established, different web browsers had varying implementations of Java.Script, leading to inconsistencies. The ECMAScript standard ensures that Java.Script behaves the same way regardless of the environment (e.g., browsers, servers).
- Continuous Improvements: ECMAScript is responsible for releasing updates to the language, adding new features, syntax, and capabilities, such as ES6 (ECMAScript 2015), which brought significant changes to Java.Script.
Think of ECMAScript as the foundation or ruleset, while Java.Script is one implementation of those rules.
3. Key Differences Between Java.Script and ECMAScript
Now that we understand what Java.Script and ECMAScript are, let’s break down the key differences between the two:
1. Definition and Scope:
- Java.Script: A scripting language used to add interactivity and dynamic features to web pages. It’s the most popular implementation of ECMAScript.
- ECMAScript: A specification that defines the standards for scripting languages like Java.Script. ECMAScript itself is not a programming language but a set of guidelines that Java.Script follows.
2. Naming and Branding:
- Java.Script: The name “Java.Script” was chosen by Netscape in 1995 to align with the growing popularity of Java, but the two languages are entirely different. The name has stuck, and Java.Script became widely adopted.
- ECMAScript: The name “ECMAScript” comes from the ECMA organization, which standardized the language to ensure consistent implementations across different browsers and platforms.
3. Updates and Versions:
- Java.Script: While “Java.Script” remains the common name, updates to the language follow the ECMAScript standard. However, developers typically refer to Java.Script features when discussing its use.
- ECMAScript: ECMAScript releases new versions that introduce features to Java.Script. For example, ES6 (2015) brought significant improvements, such as arrow functions, promises, and classes.
4. Implementations of ECMAScript:
- Java.Script is not the only implementation of ECMAScript. Other languages like JScript (used in older versions of Internet Explorer) and ActionScript (used in Adobe Flash) also followed ECMAScript specifications.
- However, Java.Script remains the most popular and widely-used implementation of ECMAScript.
4. Evolution of ECMAScript and Java.Script
The evolution of Java.Script has largely been driven by updates to the ECMAScript specification. Over the years, ECMAScript has introduced new versions, each adding significant features that enhance the functionality of Java.Script.
Major ECMAScript Versions:
- ES3 (1999): The first widely-adopted version of ECMAScript, which established Java.Script as a powerful tool for web development.
- ES5 (2009): Introduced new features like strict mode, JSON support, and additional methods for arrays and objects.
- ES6/ES2015: A landmark update that brought many modern features, including arrow functions, template literals, destructuring, and modules.
- ES7/ES2016 and Beyond: ECMAScript continues to release yearly updates, introducing features such as async/await (ES8), optional chaining (ES2020), and private class fields (ES2021).
5. Why Understanding the Difference Matters for Developers
As a developer, understanding the difference between Java.Script and ECMAScript helps you stay informed about the latest updates, features, and capabilities of the language. It’s also important to recognize that when people talk about “Java.Script,” they are often referring to the specific implementation of the ECMAScript standard.
Benefits of Knowing ECMAScript:
- Improved Coding Practices: By understanding ECMAScript updates, you can write more modern and efficient Java.Script code.
- Browser Compatibility: Knowing which ECMAScript features are supported by different browsers helps you write cross-browser compatible code.
- Leveraging Modern Features: ECMAScript constantly evolves, and knowing what new features are available can help you optimize your applications for performance, readability, and maintainability.
Java.Script vs. ECMAScript
In summary, Java.Script is a programming language that implements the ECMAScript standard. While Java.Script is the most popular and widely-used implementation, ECMAScript serves as the blueprint that defines how the language should behave across different platforms and environments.
As a web developer, it’s essential to understand this relationship so you can take full advantage of the latest ECMAScript features while building powerful and modern web applications with Java.Script. Whether you’re working with client-side interactivity, server-side programming, or full-stack development, staying up to date with ECMAScript standards will help you write cleaner, more efficient code that runs smoothly across all platforms.
Let’s See Java.Script in Action: A Beginner’s Guide
Java.Script is a powerful programming language that breathes life into websites. Whether you’re interacting with dynamic content, playing a game online, or filling out a form, Java.Script is working behind the scenes to make things interactive and engaging. In this article, we’ll explore how Java.Script works, dive into some practical examples, and see how it can enhance web development. Let’s see Java.Script in action!
1. What is Java.Script?
Java.Script is a client-side scripting language used to create dynamic content on web pages. It’s one of the three core technologies of web development, along with HTML (which structures a webpage) and CSS (which styles it). Java.Script allows developers to manipulate web pages in real time, respond to user inputs, and make websites interactive.
2. Why Java.Script is Essential for Modern Websites
Java.Script makes websites more than just static pages of text and images. It enables features like:
- Dynamic Content: Update page elements without reloading the whole page.
- Form Validation: Check if the form fields are filled out correctly before submitting.
- Animations: Add movement to web elements, making the site more engaging.
- Interactive UI: Provide better user experiences with dropdowns, modals, and more.
Without Java.Script, websites would be static and unable to offer the dynamic, user-friendly features we expect today.
3. Basic Java.Script Example: Adding Interactivity
Let’s start with a simple example of how Java.Script can make your webpage interactive. Imagine you want to display a greeting message when a button is clicked.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript in Action</title>
</head>
<body>
<h1>Click the button to see Java.Script in action!</h1>
<button onclick=“showMessage()”>Click Me</button>
<p id=“greeting”></p>
<script>
function showMessage() {
document.getElementById(‘greeting’).innerHTML = “Hello! Welcome to the world of JavaScript!”;
}
</script>
</body>
</html>
How It Works:
- HTML Structure: This sets up a button and a paragraph where the message will be displayed.
- Java.Script Function: The
showMessage()
function changes the content of the<p>
tag when the button is clicked. - Event Handling: The
onclick
attribute in the button triggers the Java.Script function when clicked.
This simple example demonstrates how Java.Script interacts with the Document Object Model (DOM) to change the content of a webpage dynamically.
4. Form Validation with Java.Script
JavaScript is widely used for validating form inputs before sending data to the server. This enhances user experience by preventing form submission errors and reducing server load.
Let’s look at a basic form validation example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Form Validation</title>
</head>
<body>
<h2>Sign Up Form</h2>
<form name=“signupForm” onsubmit=“return validateForm()”>
Name: <input type=“text” id=“name”><br><br>
Email: <input type=“email” id=“email”><br><br>
<input type=“submit” value=“Submit”>
</form>
<p id=“error”></p>
<script>
function validateForm() {
var name = document.getElementById(“name”).value;
var email = document.getElementById(“email”).value;
var error = document.getElementById(“error”);
if (name === “” || email === “”) {
error.innerHTML = “Both fields are required!”;
return false;
}
error.innerHTML = “”;
return true;
}
</script>
</body>
</html>
How It Works:
- Form Setup: The form has two input fields, one for a name and another for an email address.
- Validation Function: The
validateForm()
function checks if the fields are empty. If they are, an error message is displayed, and form submission is prevented. - OnSubmit Event: Java.Script validates the form when the user clicks the submit button.
This is a practical use of Java.Script to ensure the user fills out the necessary information before submitting the form.
5. Manipulating Styles with Java.Script
Java.Script can also be used to manipulate CSS and dynamically change the styles of HTML elements. For example, you might want to change the color of a heading when a button is clicked.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Styling</title>
</head>
<body>
<h1 id=“title”>Change my color with JavaScript!</h1>
<button onclick=“changeColor()”>Change Color</button>
<script>
function changeColor() {
document.getElementById(‘title’).style.color = “blue”;
}
</script>
</body>
</html>
How It Works:
- HTML Setup: The page contains a heading and a button.
- Style Change: The
changeColor()
function changes the color of the heading to blue when the button is clicked.
This example highlights how you can use Java.Script to interact with and change CSS properties, allowing for dynamic styling without needing to reload the page.
6. Java.Script and External APIs
One of the most exciting features of Java.Script is its ability to interact with external APIs. You can use Java.Script to fetch data from servers, display weather updates, load social media posts, or pull in live data from an API.
Here’s a basic example of fetching data from an API:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript API Example</title>
</head>
<body>
<h2>Get a Random Dog Picture</h2>
<button onclick=“getDogPicture()”>Fetch Dog Image</button>
<br><br>
<img id=“dogImage” width=“300” height=“300”>
<script>
function getDogPicture() {
fetch(‘https://dog.ceo/api/breeds/image/random’)
.then(response => response.json())
.then(data => {
document.getElementById(‘dogImage’).src = data.message;
})
.catch(error => console.error(‘Error:’, error));
}
</script>
</body>
</html>
How It Works:
- API Call: The
fetch()
function is used to make a request to the Dog CEO API, which returns a random dog image. - Display Data: Once the data is received, the image URL is inserted into the
src
attribute of the<img>
tag, displaying the dog picture.
This is a great example of how Java.Script can connect to external resources and dynamically update web content based on the data retrieved from an API.
Java.Script in Action
Java.Script is a powerful and versatile language that enables interactive, dynamic, and responsive web applications. From simple button clicks to fetching data from APIs, the possibilities with Java.Script are endless. Whether you’re validating forms, manipulating styles, or integrating with external APIs, Java.Script is the driving force behind the interactive web experiences we all rely on today.
By seeing Java.Script in action through these examples, you can start to appreciate the many ways it enhances web development and makes the web more dynamic and user-friendly. So, if you’re just starting out, take some time to practice these concepts and explore how Java.Script can make your websites more engaging and interactive! home